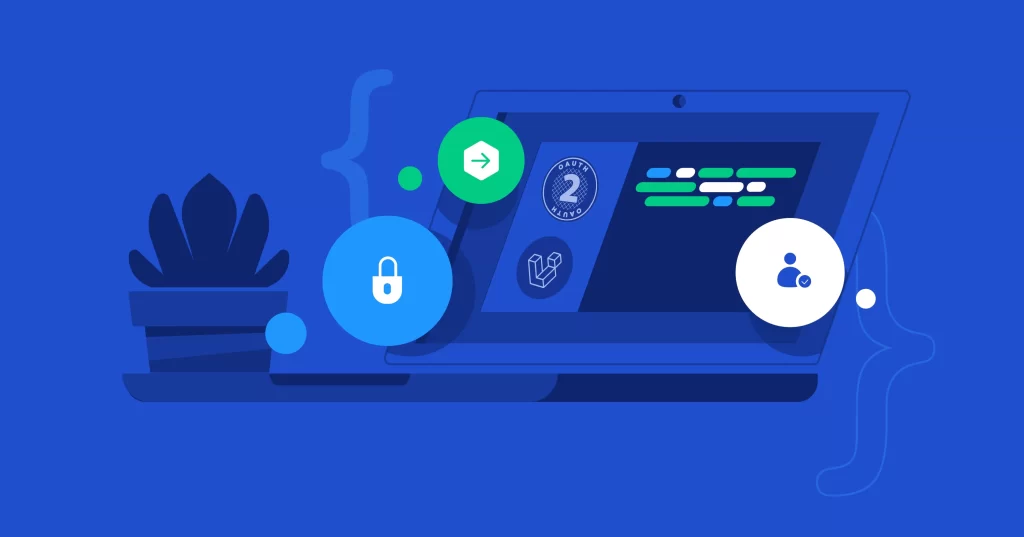
Laravel Tutorial 1
Introduction to Laravel
Laravel is a free, open-source PHP web application framework that aims to make web development easier and faster. It follows the Model-View-Controller (MVC) architectural pattern and emphasizes the use of convention over configuration. Laravel provides many built-in features, such as authentication, routing, caching, and database management, to help developers build robust and scalable applications. In this tutorial, we will cover the basics of Laravel and show you how to create a simple web application using this framework.
PREPARATION: Preparing Your Environment
Before we dive into Laravel, we need to set up our development environment. First, make sure that you have PHP installed on your computer. You can download the latest version of PHP from the official website. Next, install a web server such as Apache or Nginx. If you’re using Windows, you can install XAMPP or WAMP, which come with both PHP and Apache pre-installed. Once you have PHP and a web server installed, you can install Laravel using Composer, a dependency manager for PHP. Simply run the following command in your terminal or command prompt:
composer global require laravel/installer
.
CREATING: Creating a Basic Laravel Application
To create a new Laravel application, run the following command in your terminal or command prompt: laravel new myapp
. This will create a new directory called ‘myapp’ with all the necessary files and folders for a basic Laravel application. You can then navigate into the ‘myapp’ directory and start the development server by running the command: php artisan serve
. This will start a local development server at http://localhost:8000/
, where you can see your application in action.
STRUCTURE: Understanding Laravel’s Directory Structure
Laravel follows a specific directory structure that makes it easy to organize your code and assets. The most important directories are ‘app’, ‘bootstrap’, ‘config’, ‘database’, ‘public’, ‘resources’, ‘routes’, and ‘tests’. The ‘app’ directory contains all the application logic, such as models, controllers, and services. The ‘bootstrap’ directory contains the code that initializes the application, such as loading the environment variables and registering service providers. The ‘config’ directory contains all the configuration files for the application, such as database settings and logging options. The ‘public’ directory contains the front-facing assets, such as images, JavaScript, and CSS files. The ‘resources’ directory contains the views, which are the HTML templates that are rendered by the application. The ‘routes’ directory contains all the route definitions for the application, which map URLs to controllers. The ‘tests’ directory contains all the unit tests for the application.
ROUTING: Routing in Laravel
Routing is the process of mapping URLs to controller actions in Laravel. To define a route, you can use the Route
facade provided by Laravel. For example, to define a route that maps to the HomeController@index
method, which renders the home page, you can use the following code:
Route::get('/', 'HomeController@index');
This code tells Laravel to respond to the root URL, i.e., http://localhost:8000/
, with the HomeController@index
method. You can also define routes for other HTTP verbs, such as POST
, PUT
, and DELETE
, using the Route::post()
, Route::put()
, and Route::delete()
methods, respectively.
VIEW: Building Your First View and Controller in Laravel
To build a view in Laravel, you can use the Blade templating engine, which provides a simple and intuitive syntax for writing HTML templates. To create a new view, simply create a new file in the ‘resources/views’ directory with a .blade.php
extension. For example, to create a view called ‘welcome.blade.php’, you can use the following code:
Welcome to My App
Hello, {{ $name }}!
This code defines a simple HTML page with a heading that displays the value of the $name
variable. To render this view, you need to define a controller method that returns the view. For example, to define a HomeController@index
method that returns the ‘welcome’ view with the name ‘John’, you can use the following code:
namespace AppHttpControllers;
use IlluminateHttpRequest;
class HomeController extends Controller
{
public function index()
{
$name = 'John';
return view('welcome', compact('name'));
}
}
This code defines a HomeController
class that extends the Controller
class provided by Laravel. The index()
method returns the ‘welcome’ view with the $name
variable passed as a parameter using the compact()
function.
Conclusion
In this tutorial, we covered the basics of Laravel and showed you how to create a simple web application using this framework. We discussed how to prepare your environment, create a basic Laravel application, understand Laravel’s directory structure, define routes in Laravel, and build your first view and controller in Laravel. With this knowledge, you should be able to start building more complex web applications using Laravel. For more information, you can check out the official Laravel documentation and the various tutorials and courses available online.
Table of Contents