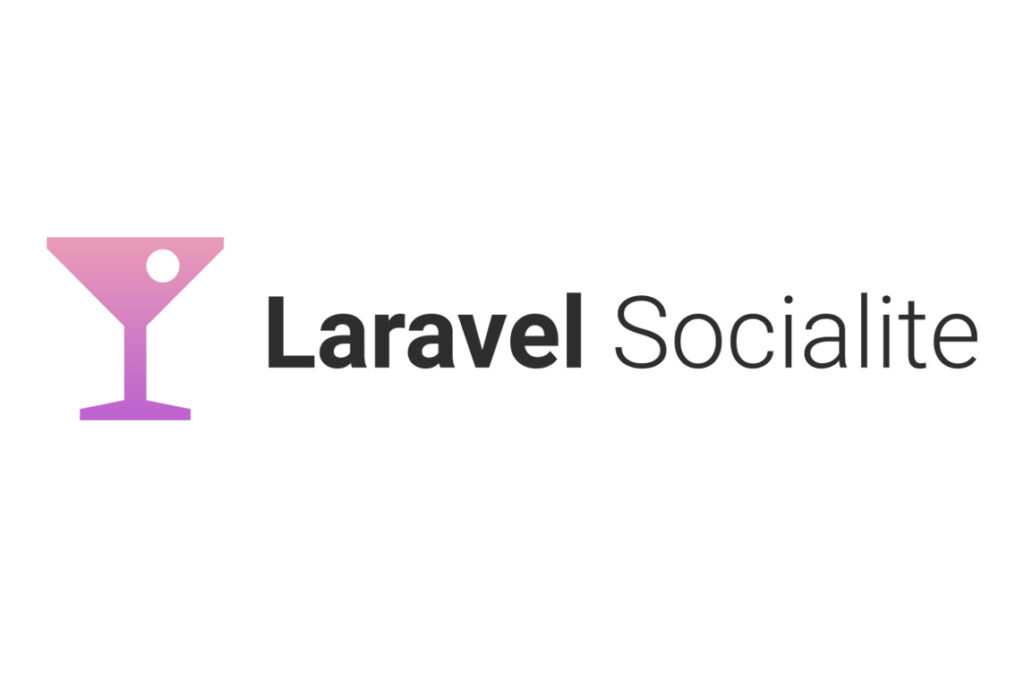
Laravel Socialite is a popular package that simplifies authentication for your Laravel applications. It allows users to log in to your application with their social media accounts such as Facebook, Twitter, and Google. This package is easy to install and use, making it a popular choice for developers. In this article, we will explore Laravel Socialite in detail.
Introduction to Laravel Socialite
Laravel Socialite is a package that provides an easy way to authenticate with OAuth providers. It allows you to authenticate users with their social media accounts. It does so by using the OAuth protocol. OAuth is an open authorization protocol that allows secure API authorization in a simple and standard method from desktop and web applications.
How Laravel Socialite Works
Laravel Socialite works by providing a simple API that allows you to authenticate users with their social media accounts. It does so by following the OAuth protocol. First, a user logs in with their social media account. Then, Laravel Socialite obtains an access token from the social media provider. This access token is used to make API requests to the social media provider’s API to get the user’s profile information.
Advantages of Using Laravel Socialite
Using Laravel Socialite has several advantages. It simplifies the authentication process, making it easy for users to log in to your application. It also saves you time by reducing the amount of code you need to write to implement authentication. Laravel Socialite provides a simple interface that abstracts away the complexities of OAuth authentication. Additionally, it allows you to integrate with multiple providers, giving your users different options to choose from.
Examples of Laravel Socialite in Action
Here are some examples of how Laravel Socialite can be used in practice:
Signing in with Facebook
To enable Facebook authentication, you need to install the Laravel Socialite package by running the following command:
composer require laravel/socialite
Then, add the following configuration settings to your .env file:
FACEBOOK_CLIENT_ID=
FACEBOOK_CLIENT_SECRET=
FACEBOOK_REDIRECT_URL=
After that, you can add the following code to your login controller:
return Socialite::driver('facebook')->redirect();
This code will redirect the user to Facebook’s login page. After the user logs in, Laravel Socialite will obtain an access token and redirect the user back to your application.
Signing in with Google
To enable Google authentication, you need to install the Laravel Socialite package and add the following configuration settings to your .env file:
GOOGLE_CLIENT_ID=
GOOGLE_CLIENT_SECRET=
GOOGLE_REDIRECT_URL=
After that, you can add the following code to your login controller:
return Socialite::driver('google')->redirect();
This code will redirect the user to Google’s login page. After the user logs in, Laravel Socialite will obtain an access token and redirect the user back to your application.
Signing in with Twitter
To enable Twitter authentication, you need to install the Laravel Socialite package and add the following configuration settings to your .env file:
TWITTER_CLIENT_ID=
TWITTER_CLIENT_SECRET=
TWITTER_REDIRECT_URL=
After that, you can add the following code to your login controller:
return Socialite::driver('twitter')->redirect();
This code will redirect the user to Twitter’s login page. After the user logs in, Laravel Socialite will obtain an access token and redirect the user back to your application.
OUTRO:
In conclusion, Laravel Socialite is a powerful authentication package that simplifies OAuth authentication with social media providers. It provides an easy-to-use interface that abstracts away the complexities of OAuth authentication. Using Laravel Socialite can save you time and help you provide your users with a smooth authentication experience.
Table of Contents